Prefill checkout properties
Prefill checkout fields to save customers time and increase checkout conversion
You can prefill properties on a checkout for a smoother checkout experience for your customer. You might do this when:
- You capture some information about a prospect on the page before they interact with your checkout.
- You've built an integration with a CRM solution that passes email or other information as parameters on signup links.
- You're working with a logged-in customer, presenting them with upgrade options.
How it works
Paddle Checkout is optimized for conversion, asking customers for:
- Email address
- Country
- ZIP/postal code, or region (only in some markets)
- Payment details
Customers can also add a discount and information about their business, like a VAT or tax number.
You can pass data to a checkout to prefill properties, reducing purchase friction for customers and increasing conversion. You can prefill all customer details. You can't prefill payment details, but you can present saved payment methods for returning customers.
Prefilling works with both overlay checkout and inline checkout. You can use HTML data attributes or JavaScript properties.
Before you begin
You'll need to include Paddle.js on your page and pass a client-side token.
To open a checkout, you'll need to create products and prices and pass them to a checkout.
Get a step-by-step overview of how to build a complete checkout — including passing checkout settings. See: Build an overlay checkout or build an inline checkout
Pass customer or business information
In this example, there's a signup button that includes an email address field. The page has a country selector.
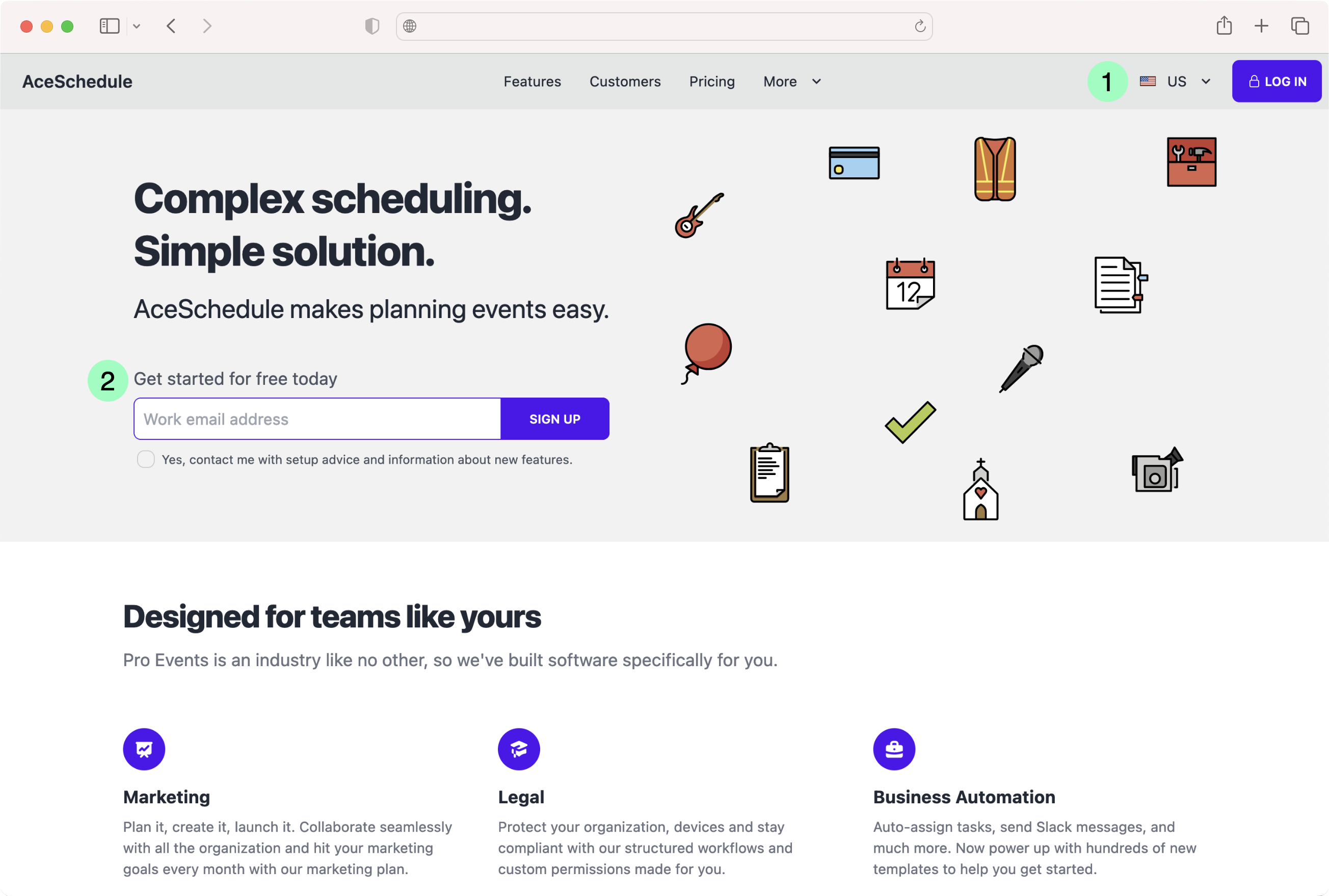
You could use HTML attributes or JavaScript properties to prefill this information in checkout:
# | Description | HTML attribute | JavaScript property |
---|---|---|---|
1 | Country | data-customer-address-country-code | customer.address.countryCode |
2 | Email address | data-customer-email | customer.email |
Add data attributes to your checkout trigger to prefill those values on a checkout.
123456789101112131415161718191<a
2 href='#'
3 class='paddle_button'
4 data-theme='light'
5 data-customer-address-country-code='US'
6 data-customer-email='jo@example.com'
7 data-items='[
8 {
9 "priceId": "pri_01gs59hve0hrz6nyybj56z04eq",
10 "quantity": 1
11 },
12 {
13 "priceId": "pri_01gs59p7rcxmzab2dm3gfqq00a",
14 "quantity": 1
15 }
16 ]'
17>
18 Buy Now
19</a>
For a full list of fields you can prefill, see: HTML data attributes
Pass customer, address, and business IDs
Instead of passing customer email, address, and business information, you can pass an existing customer ID, address ID, or business ID.
You might do this if you're working with a logged-in customer who's looking to upgrade or purchase another subscription, or if you have a CRM integration that creates entities in Paddle for prospects.
Customer ID, address ID, and business ID replace other customer, address, and business fields. For example, you should pass either customer ID or customer email — not both.
Add data attributes to your checkout trigger to prefill those values on a checkout.
12345678910111213141516171819201<a
2 href='#'
3 class='paddle_button'
4 data-theme='light'
5 data-customer-id='ctm_01gm82kny0ad1tk358gxmsq87m'
6 data-customer-address-id='add_01gm82v81g69n9hdb0v9sw6j40'
7 data-business-id='biz_01gnymqsj1etmestb4yhemdavm'
8 data-items='[
9 {
10 "priceId": "pri_01gm81eqze2vmmvhpjg13bfeqg",
11 "quantity": 1
12 },
13 {
14 "priceId": "pri_01gm82kny0ad1tk358gxmsq87m",
15 "quantity": 1
16 }
17 ]'
18>
19 Buy Now
20</a>
For a full list of fields that you can prefill, see: HTML data attributes
Apply a discount
You can pass the Paddle ID of a discount entity or its discount code to a checkout to automatically apply it — no need for customers to enter a code.
enabled_for_checkout
must betrue
against the discount entity to apply it to a checkout.
Add data attributes to your checkout trigger to prefill those values on a checkout.
12345678910111213141516171819201<a href='#'
2 class='paddle_button'
3 data-display-mode='overlay'
4 data-theme='light'
5 data-locale='en'
6 data-items='[
7 {
8 "priceId": "pri_01gm81eqze2vmmvhpjg13bfeqg",
9 "quantity": 1
10 },
11 {
12 "priceId": "pri_01gm82kny0ad1tk358gxmsq87m",
13 "quantity": 1
14 },
15 {
16 "priceId": "pri_01gm82v81g69n9hdb0v9sw6j40",
17 "quantity": 1
18 }
19 ]'
20 data-discount-id='dsc_01gp0ynsntfpyw2spd2md1wqx1'
See: HTML data attributes
Build a one-page checkout
One-page checkout experience
Pass variant
with the value one-page
as a checkout setting to present customers with a one-page checkout experience. Paddle Checkout collects customer information and payment details on the same page.
1234567891011121314151617181var itemsList = [
2 {
3 priceId: 'pri_01gm81eqze2vmmvhpjg13bfeqg',
4 quantity: 1
5 },
6 {
7 priceId: 'pri_01gm82kny0ad1tk358gxmsq87m',
8 quantity: 1
9 }
10];
11
12Paddle.Checkout.open({
13 settings: {
14 displayMode: "overlay",
15 variant: "one-page"
16 },
17 items: itemsList,
18});
Multi-page checkout
If you prefer the multi-page checkout experience, Paddle skips the first page of checkout when all required fields are prefilled. This means customers land on a screen where all they need to do is enter their payment details.
To jump to the second page on the multi-page inline checkout, prefill either required properties or Paddle IDs:
Description | HTML attribute | JavaScript property |
---|---|---|
Country | data-customer-address-country-code | customer.address.countryCode |
Email address | data-customer-email | customer.email |
ZIP/postal code (only where required) | data-customer-address-postal-code | customer.address.postalCode |
Region (only for United Arab Emirates) | data-customer-address-region | customer.address.region |